2 marks Question of Programming for Problem Solving – Unit 1
In this blog we cover 2 marks Question of Programming for Problem Solving from Unit 1. Here we try to cover all possible question from this unit
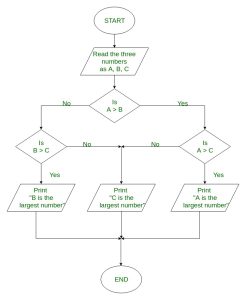
Q2 Write a function to interchange the two values of two variables without using third variable?
Q3 Differentiate complier and interpreter?
Q4 #define PRODUCT(n) n*n
- Clarity: The algorithm should be clear and unambiguous so that it can be easily understood and followed by anyone who reads it.
- Input and output: The algorithm should have well-defined input and output parameters, which should be clearly stated at the beginning of the algorithm.
- Correctness: The algorithm should produce the correct output for all valid inputs. It should solve the problem it is intended to solve, without errors or bugs.
- Finiteness: The algorithm should terminate after a finite number of steps. It should not run indefinitely or enter an infinite loop.
- Efficiency: The algorithm should be efficient and run in a reasonable amount of time, given the size and complexity of the problem it is solving.
- Generality: The algorithm should be general enough to handle a wide range of inputs, and not be specific to a single problem or input.
- Modularity: The algorithm should be modular, meaning that it can be broken down into smaller, more manageable parts that can be tested and optimized separately.
- Reusability: The algorithm should be designed in such a way that it can be easily adapted and reused for similar problems or tasks.
- Robustness: The algorithm should be able to handle unexpected or invalid inputs in a graceful manner, without crashing or producing incorrect output.
- Maintainability: The algorithm should be designed in such a way that it can be easily updated, modified, or extended in the future, as new requirements arise or as the problem evolves.
Q6 What do you mean by scope and life time of a variable?
- Scope of a variable: The scope of a variable is the portion of the program where the variable is visible and can be accessed. In other words, it determines where the variable can be used in the program. The scope of a variable is defined by the block of code in which the variable is declared. In C, for example, variables declared inside a function have a local scope and can only be accessed within that function, while variables declared outside any function have a global scope and can be accessed from anywhere in the program.
- Lifetime of a variable: The lifetime of a variable is the period of time during which the variable exists and retains its value. In other words, it determines how long the variable remains in memory. The lifetime of a variable is also determined by its scope. Local variables have a shorter lifetime than global variables. When a function is called, its local variables are created and allocated memory, and when the function returns, the local variables are destroyed and their memory is freed. Global variables, on the other hand, exist for the entire duration of the program.
Q7 What do you mean by precedence and associativity while solving some arithmetic expressions?
- Precedence:Precedence determines the order in which operators are evaluated in an expression. Operators with higher precedence are evaluated before operators with lower precedence. For example, in the expression 4 + 5 * 3, the multiplication operator * has a higher precedence than the addition operator +, so it is evaluated first. Therefore, the expression is evaluated as 4 + (5 * 3), which results in the value 19.
- Associativity: Associativity determines the order in which operators of the same precedence are evaluated. Operators can be left-associative or right-associative. Left-associative operators are evaluated from left to right, while right-associative operators are evaluated from right to left. For example, in the expression 9 – 3 – 2, both subtraction operators have the same precedence and are left-associative. Therefore, the expression is evaluated as (9 – 3) – 2, which results in the value 4.
Q8 draw the memory hierarchical structure of computer system?
- Registers: This is the fastest and smallest type of memory in a computer system, consisting of a few dozen or hundred bytes of storage that are integrated into the CPU. Registers are used to hold data that is being actively processed by the CPU, such as instructions, data operands, and memory addresses.
- Cache: This is a type of high-speed memory that sits between the registers and the main memory. Caches are used to store copies of frequently accessed data from the main memory, which can be accessed faster than reading the data from the main memory. Caches are typically divided into several levels, with each level having a larger capacity but slower access time than the previous level.
- Main Memory: This is the primary memory in a computer system, also known as RAM (Random Access Memory). It provides a large, fast storage area for the operating system and applications to store data and program instructions. The main memory is volatile, meaning that its contents are lost when the power is turned off.
- Secondary Storage: This includes non-volatile storage devices such as hard disk drives (HDDs), solid-state drives (SSDs), and optical drives. These devices provide a large and relatively slow storage area for storing data and programs that are not currently in use. Secondary storage devices have much larger capacity than main memory but are slower to access.
- Tertiary Storage: This includes long-term, off-line storage devices such as magnetic tape and optical disks. These devices are used for archival storage and backup purposes and have very slow access times compared to other types of memory.
Overall, the memory hierarchy is designed to balance the need for fast access to data with the cost and capacity of different types of memory. By using a combination of fast, expensive memory and slower, cheaper memory, computer systems can provide the best possible performance at a reasonable cost.
- Memory Management: An OS manages the allocation and deallocation of memory to running processes and ensures that each process has access to the required amount of memory.
- Process Management: An OS manages the execution of processes or programs, including their creation, scheduling, and termination.
- File Management: An OS manages the creation, deletion, and organization of files and directories, and provides mechanisms for accessing and manipulating file data.
- Device Management: An OS manages input and output (I/O) devices, such as keyboards, mice, printers, and network adapters, and provides a consistent interface for accessing these devices.
- Security:An OS provides mechanisms to protect the system and user data from unauthorized access and ensure the privacy and integrity of information.
- Networking: An OS provides networking support to allow communication between computers, including protocols for transferring data, managing connections, and handling errors.
- User Interface: An OS provides a user-friendly interface to interact with the computer system, including graphical user interfaces (GUIs), command-line interfaces (CLIs), and touch-based interfaces.
- Error Handling: An OS detects and handles errors that occur in the system, including software errors, hardware errors, and user errors.
- Resource Allocation:An OS manages system resources, including CPU time, memory, and I/O bandwidth, to ensure that all processes have a fair share of available resources.
- System Monitoring: An OS monitors system performance and generates statistics and reports to help administrators manage the system and troubleshoot problems
Q11 Write a function in C to exchange the content of two integer variables without using a third variable?
- Start/End: This symbol represents the beginning or end of a process and is typically represented by an oval shape.
- Process: This symbol represents a specific operation or action performed in the process and is typically represented by a rectangular shape.
- Decision: This symbol represents a decision point in the process, where the flow can take different paths based on a condition. It is typically represented by a diamond shape.
- Input/Output: This symbol represents the input or output of data in the process and is typically represented by a parallelogram shape.
- Connector:This symbol represents a point where the flowchart connects to another part of the process or another page in the flowchart. It is typically represented by a small circle.
- Flowlines: These lines connect the symbols and show the flow of the process.
Q14 Write an algorithm to find the greatest number among three numbers?
- Start
- Input three numbers a, b, c
- Set a variable max to a
- If b > max, set max to b
- If c > max, set max to c
- Output max as the greatest number
- End
- The algorithm starts.
- The user inputs three numbers a, b, and c.
- The variable max is initialized to a, assuming that a is the greatest number.
- If b is greater than max, then max is set to b.
- If c is greater than max, then max is set to c.
- The value of max is the greatest number, so it is outputted.
- The algorithm ends.
Q15 What is meant by storage classes of a variable?
- Automatic Storage Class: This is the default storage class for variables declared inside a function. Variables declared with this storage class are created when the function is called and destroyed when the function exits. The initial value of automatic variables is garbage.
- Static Storage Class: Variables declared with this storage class have a lifetime throughout the execution of the program. They are initialized only once, at the beginning of the program, and retain their values until the program terminates. Static variables can only be accessed within the scope they are declared.
- Register Storage Class: This storage class is used to declare variables that will be stored in the CPU registers instead of memory. The number of CPU registers available is limited, so the use of register variables should be limited to frequently accessed variables to improve performance.
- Extern Storage Class: This storage class is used to declare variables that are defined in another file or module. It is used when a variable needs to be shared between different files or modules in a program.
Q16 What is token in ‘C’ language?
In the C programming language, a token is the smallest unit in the source code. C programs are made up of various tokens, which serve as building blocks for writing code. Tokens can be classified into several categories:
- Keywords: Keywords are reserved words in C that have special meanings. Examples include int, if, else, for, while, etc.
- Identifiers: Identifiers are used to name variables, functions, arrays, etc., in a C program. An identifier can be composed of letters, digits, and underscores, but it cannot start with a digit. For example, sum, counter, and total Amount are identifiers.
- Constants: Constants are literal values that do not change during program execution. They can be of various types, such as integer constants (5, 10), floating-point constants (3.14), character constants (‘A’, ‘b’), or string constants (“Hello, World!”).
- String literals: String literals are sequences of characters enclosed within double quotes, like “Hello, World!”.
- Operators: Operators perform operations on variables and values. Examples include +, -, *, /, and %.
- Punctuation symbols: Punctuation symbols like , (comma), ; (semicolon), { (left curly brace), } (right curly brace), ( (left parenthesis), and ) (right parenthesis) are used to structure the code.
- Special symbols: Special symbols include # (hash), = (assignment), == (equality), and != (not equal), among others.
During the compilation process, the C compiler breaks down the source code into these tokens, which are then used for syntax analysis and further processing to generate executable code. Understanding tokens is essential for writing correct and meaningful C programs.
In C programming, formatted output refers to the process of printing data on the screen or other output devices in a specific, well-formatted manner. The printf() function is commonly used for formatted output in C. It allows you to print variables and literal strings while specifying the format in which the data should be displayed.
Here’s the basic syntax of the printf() function:
printf(“format string”, variable1, variable2, …);
In the format string, placeholders are used to specify the type and format of the variables you want to print. For example:
%d is used for integers
%f is used for floating-point numbers
%c is used for characters
%s is used for strings
Let’s look at an example to illustrate formatted output in C:
#include <stdio.h>
int main() {
int num = 42;
float pi = 3.14159;
char ch = ‘A’;
char name[] = “John”;
// Printing formatted output
printf(“Integer: %d\n”, num);
printf(“Float: %.2f\n”, pi); // %.2f limits the floating-point output to 2 decimal places
printf(“Character: %c\n”, ch);
printf(“String: %s\n”, name);
return 0;
}
In this example, the printf() function is used to print the integer num, the floating-point number pi with two decimal places, the character ch, and the string name. The format specifiers (%d, %.2f, %c, %s) are replaced by the corresponding values during execution, producing a well-formatted
Output:
Integer: 42
Float: 3.14
Character: A
String: John
Formatted output is essential for displaying data in a readable and organized manner, especially when dealing with complex programs where various data types need to be presented clearly to the user.